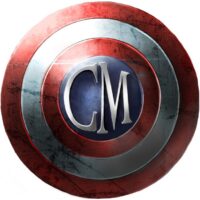
WordPress is by far the number one website framework in the world. It’s very well coded and can be infinitely customized to meet any requirements for any online business.
Below are a few customizations I’ve built over the years to help improve client sites. Please keep in mind that I’ve been working with WordPress since 2009, so some of these customizations may not work with your version of WordPress, while others have been updated to only work with newer versions of WordPress.
Please know that any of these customization’s could disable some part of the website that’s required to make the site function properly. Use these modifications with caution and be sure to test, test, test.
Most of these code snippets will be posted in the functions.php file. The minimum PHP version is 7.0.
Of course, any of these code snippets can be added to a custom plugin, or inserted into any number of WordPress plugins that allow for custom code.
These are advanced code snippets that require some knowledge of how to implement this code inside your WordPress environment. Please use with caution or look me up on Upwork.
// CODEMILITANT - DISABLE EMOJIS AND WASTEFUL CONTENT
remove_action( 'wp_head', 'rsd_link' );
remove_action( 'wp_head', 'wlwmanifest_link' );
remove_action( 'wp_head', 'wp_generator' );
remove_action( 'wp_head', 'wc_generator' );
remove_action( 'wp_head', 'print_emoji_detection_script', 7 );
remove_action( 'admin_print_styles', 'print_emoji_styles' );
remove_action( 'admin_print_scripts', 'print_emoji_detection_script' );
remove_action( 'wp_print_styles', 'print_emoji_styles' );
remove_action( 'wp_head', 'wp_resource_hints', 2 );
remove_filter( 'the_content_feed', 'wp_staticize_emoji' );
remove_filter( 'comment_text_rss', 'wp_staticize_emoji' );
remove_filter( 'embed_head', 'print_emoji_detection_script' );
remove_filter( 'wp_mail', 'wp_staticize_emoji_for_email' );
add_filter( 'option_use_smilies', '__return_false' );
//CODEMILITANT - MOVE JAVASCRIPTS TO FOOTER
remove_action('wp_head', 'wp_print_scripts');
remove_action('wp_head', 'wp_print_head_scripts', 9);
add_action('wp_footer', 'wp_print_scripts', 5);
add_action('wp_footer', 'wp_print_head_scripts', 5);
//CODEMILITANT - REMOVE STRIPE JAVASCRIPTS FROM PRODUCT PAGES
add_filter( 'wc_stripe_load_scripts_on_product_page_when_prbs_disabled', '__return_false' );
add_filter( 'wc_stripe_load_scripts_on_cart_page_when_prbs_disabled', '__return_false' );
// CODEMILITANT - REMOVE WOOCOMMERCE MINI CART
add_filter( 'woocommerce_widget_cart_is_hidden', '__return_true' );
//CODEMILITANT: DESIGNATE THUMBNAIL SIZES
function cm_thumbnail_setup() {
add_theme_support( 'post-thumbnails' );
set_post_thumbnail( get_the_ID(), '20' );
set_post_thumbnail_size( 200, 200 );
}
add_action( 'after_setup_theme', 'cm_thumbnail_setup' );
// CODEMILITANT - REDIRECT ALL 404 URLS FROM POSTNAME TO CATEGORY/POSTNAME
function cm_redirect_to_category( $template ) {
if ( ! is_404() )
return $template;
global $wp_rewrite, $wp_query;
if ( '/%category%/%postname%/' !== $wp_rewrite->permalink_structure )
return $template;
if ( ! $post = get_page_by_path( $wp_query->query['category_name'], OBJECT, 'post' ) )
return $template;
$permalink = get_permalink( $post->ID );
wp_redirect( $permalink, 301 );
exit;
}
add_filter( '404_template', 'cm_redirect_to_category' );
//CODEMILITANT - REQUIRE FIRST AND LAST NAME IN USER PROFILE
function cm_require_first_last( $errors, $update, $user ) {
if ( is_admin() ) {
// Use the $_POST variable to check required fields
if( empty($_POST['first_name']) )
// add an error message to the WP_Errors object
$errors->add( 'first_name_required',__('First name is required.') );
if( empty($_POST['last_name']) )
// add an error message to the WP_Errors object
$errors->add( 'last_name_required',__('Last name is required.') );
}
}
add_filter( 'user_profile_update_errors', 'cm_require_first_last', 10, 3 );
//CODEMILITANT - ADD AND REQUIRE NEW CHECKOUT FIELDS
function cm_custom_checkout_fields($fields) {
$fields['billing']['fqdn_domain']['label'] = "FQDN Domain [yourdomain.com with no www]";
$fields['billing']['fqdn_domain']['required'] = true;
$fields['billing']['fqdn_domain']['placeholder'] = 'yourdomain.com';
return $fields;
}
add_filter('woocommerce_checkout_fields', 'cm_custom_checkout_fields');
// CODEMILITANT REMOVE CART/CHECKOUT NOTICES
add_filter( 'woocommerce_coupon_message', '__return_false' );
//CODEMILITANT - MINIMUM COMMENT LENGTH REQUIRED
function minimal_comment_length( $commentdata ) {
$minimalCommentLength = 120;
if ( strlen( trim( $commentdata['comment_content'] ) ) < $minimalCommentLength ){ wp_die( 'All comments must be at least ' . $minimalCommentLength . ' characters long.' ); } return $commentdata; } add_filter( 'preprocess_comment', 'minimal_comment_length' );
//CODEMILITANT - MINIMUM COMMENT LENGTH REQUIRED
function minimal_comment_length( $commentdata ) {
$minimalCommentLength = 120;
if ( strlen( trim( $commentdata['comment_content'] ) ) < $minimalCommentLength ){ wp_die( 'All comments must be at least ' . $minimalCommentLength . ' characters long.' ); } return $commentdata; } add_filter( 'preprocess_comment', 'minimal_comment_length' );
//CODEMILITANT - DISABLE LAZY LOADING
add_filter( 'wp_lazy_loading_enabled', '__return_false' );
//CODEMILITANT - MODIFIED ROBOTS TXT FILE function cm_update_robots_txt( $output, $public ) { $output = ""; $output .= "# Allow Wanted Bots\n"; $output .= "User-agent: *\n"; $output .= "Allow: /\n"; $output .= "Disallow: /wp-admin/\n"; $output .= "Allow: /wp-admin/admin-ajax.php\n"; $output .= "Allow: /ads.txt\n\n"; $output .= "# Disallow Unwanted Bots\n"; $output .= "User-agent: DotBot\n"; $output .= "User-agent: SemrushBot\n"; $output .= "User-agent: PetalBot\n"; $output .= "User-agent: YextBot\n"; $output .= "User-agent: AhrefsBot\n"; $output .= "User-agent: YandexBot\n"; $output .= "User-agent: DataForSeoBot\n"; $output .= "User-agent: Slurp\n"; $output .= "User-agent: MJ12Bot\n"; $output .= "Disallow: /\n"; $new_wp_sitemap = new WP_Sitemaps(); if ( $public ) $output .= "\nSitemap: " . esc_url( $new_wp_sitemap->index->get_index_url() ) . "\n";
return $output;
}
add_filter( 'robots_txt', 'cm_update_robots_txt', 10, 2 );
//CODEMILITANT - RUN WORDPRESS OVER HTTP (NON-SSL)
define('FORCE_SSL_ADMIN', true);
if (strpos($_SERVER['HTTP_X_FORWARDED_PROTO'], 'https') !== false)
$_SERVER['HTTPS']='on';
// VERIFY WP-CRON CAN PERFORM A LOOPBACK REQUEST
$url = site_url( 'wp-cron.php' );
$response = wp_remote_post( $url, compact( 'body', 'cookies', 'headers', 'timeout', 'sslverify' ) );
foreach( $response as $res ) {
echo "$res";
}
//CODEMILITANT - CREATE CUSTOM CANONICAL URLS
remove_action('wp_head', 'rel_canonical');
function cm_rel_canonical() {
if (is_singular('post') && isset($_GET['pubID'])) {
global $post;
$link = get_permalink($post->ID) . '?pubID=' . absint($_GET['pubID']);
echo "<link rel='canonical' href='$link' />\n";
} else {
rel_canonical();
}
}
add_action('wp_head', 'cm_rel_canonical');
//CODEMILITANT - TEST MEMCACHED SERVER STATUS
$key = 'dummy';
$value = '100';
$dummy_value = wp_cache_get( $key );
if ( $value !== $dummy_value ) {
echo "The dummy value is not in cache. Adding the value now.";
wp_cache_set( $key, $value );
} else {
echo "Value is " . $dummy_value . ". The WordPress Memcached Backend is working!";
}
//PUT THIS IN WP-CONFIG.PHP
global $memcached_servers;
$memcached_servers = array( array('127.0.0.1', 11211, 0) );
//THIS REQUIRES THE GITHUB TOLLMANZ WORDPRESS PECL MEMCACHED OBJECT CACHE
https://github.com/tollmanz/wordpress-pecl-memcached-object-cache
//BE SURE TO PLACE THE "object-cache.php" FILE INSIDE THE WP-CONTENT FOLDER
// CODEMILITANT - DECREASE BIG IMAGE THRESHOLD DOWN TO 1200
function cm_big_image_size_render( $threshold ) {
return 1200;
}
add_filter('big_image_size_threshold', 'cm_big_image_size_render', 999, 1);
// CODEMILITANT: DISABLE IMAGE THRESHOLD RESIZE
add_filter( 'big_image_size_threshold', '__return_false' );
//CODEMILITANT ENQUEUE LOCAL FONTS
function cm_local_fonts() {
$query_args = array(
'family' => 'Source+Sans+Pro:200,200italic,300,300italic,regular,italic,600,600italic,700,700italic,900,900italic|Lora:regular,italic,700,700italic',
'subset' => 'cyrillic,cyrillic-ext,latin,latin-ext'
);
wp_register_style( 'cm_local_fonts', add_query_arg( $query_args, '/static/fonts/' ), array(), null );
wp_enqueue_style( 'cm_local_fonts', add_query_arg( $query_args, '/static/fonts/' ), array(), null );
}
add_action('wp_enqueue_scripts', 'cm_local_fonts');
//CODEMILITANT - REMOVE CSS AND JS FROM SPECIFIED NON WOOCOMMERCE PAGES
function cm_disable_loading_css_js() {
if ( function_exists('WC') && ! is_admin() ) { //must be here in the event woocommerce plugin is not activated/installed
if ( is_front_page() || is_singular('post') ) {
// Dequeue TwentyTwenty styles
wp_dequeue_style( 'twentytwenty-print-style' );
// Dequeue Woocommerce styles
wp_dequeue_style( 'woocommerce-smallscreen' );
wp_dequeue_style( 'woocommerce-layout' );
wp_dequeue_style( 'woocommerce-general' );
wp_deregister_style( 'wc-blocks-vendors-style' );
wp_dequeue_style( 'wc-blocks-vendors-style' );
wp_deregister_style( 'wc-blocks-style' );
wp_dequeue_style( 'wc-blocks-style' );
wp_deregister_style( 'acfw-blocks-frontend' );
wp_dequeue_style( 'acfw-blocks-frontend' );
wp_deregister_style( 'berocket_aapf_widget-style' );
wp_dequeue_style( 'berocket_aapf_widget-style' );
// Dequeue Woocommerce scripts
wp_deregister_script( 'woocommerce' );
wp_dequeue_script( 'woocommerce' );
wp_deregister_script( 'jquery-blockui' );
wp_dequeue_script( 'jquery-blockui' );
wp_deregister_script( 'js-cookie' );
wp_dequeue_script( 'js-cookie' );
wp_deregister_script( 'ppcp-smart-button' );
wp_dequeue_script( 'ppcp-smart-button' );
wp_deregister_script( 'mailchimp-woocommerce' );
wp_dequeue_script( 'mailchimp-woocommerce' );
// Dequeue Share Cart Styles
wp_dequeue_style( 'share-cart-url-for-woo' );
// Dequeue Share Cart Javascripts
wp_deregister_script( 'model-js' );
wp_dequeue_script( 'model-js' );
wp_deregister_script( 'jb-js' );
wp_dequeue_script( 'jb-js' );
wp_deregister_script( 'share-cart-url-for-woo-js' );
wp_dequeue_script( 'share-cart-url-for-woo-js' );
}
}
}
add_action( 'wp_enqueue_scripts', 'cm_disable_loading_css_js', 100 );
// CODEMILITANT - SERVE ALL CSS/JS AND IMAGES OVER HTTPS
add_filter('script_loader_src', 'cm_agnostic_script_loader_src', 20, 2);
function cm_agnostic_script_loader_src($src, $handle) {
return preg_replace('/^(http|https):/', '', $src);
}
add_filter('style_loader_src', 'cm_agnostic_style_loader_src', 20, 2);
function cm_agnostic_style_loader_src($src, $handle) {
return preg_replace('/^(http|https):/', '', $src);
}
add_filter('wp_get_attachment_url', 'cm_agnostic_image_loader', 20);
function cm_agnostic_image_loader($url) {
return preg_replace('/^(http|https):/', '', $url);
}
// CODEMILITANT - PRIVATIZE WORDPRESS WEBSITE
function cm_make_wordpress_site_private() {
if ( (is_page('1150') || is_page('7')) )
return;
if ( ! is_user_logged_in() ) {
$redirect = home_url() . '/my-account/?redirect_to=https://' . $_SERVER["HTTP_HOST"] . urlencode($_SERVER["REQUEST_URI"]);
wp_redirect( esc_url($redirect) );
exit;
}
}
add_action('wp', 'cm_make_wordpress_site_private');
// CODEMILITANT - ADD ADDIITONAL COLUMNS TO WOOCOMMERCE ORDERS
// ADDING 2 NEW COLUMNS WITH THEIR TITLES (keeping "Total" and "Actions" columns at the end)
add_filter( 'manage_edit-shop_order_columns', 'custom_shop_order_column', 20 );
function custom_shop_order_column($columns) {
$reordered_columns = array();
// Inserting columns to a specific location
foreach( $columns as $key => $column) {
$reordered_columns[$key] = $column;
if( $key == 'order_status' ) {
// Inserting after "Status" column
$reordered_columns['po-column'] = __( 'PO Number','theme_domain');
// $reordered_columns['my-column2'] = __( 'Title2','theme_domain');
}
}
return $reordered_columns;
}
// Adding custom fields meta data for each new column
add_action( 'manage_shop_order_posts_custom_column' , 'custom_orders_list_column_content', 20, 2 );
function custom_orders_list_column_content( $column, $post_id ) {
switch ( $column ) {
case 'po-column' :
// Get custom post meta data
$po_number = get_post_meta( $post_id, '_po_number', true );
if(!empty($po_number))
echo $po_number;
else
echo '<small>(<em>No PO value</em>)</small>';
break;
/* case 'my-column2' :
// Get custom post meta data
$my_var_two = get_post_meta( $post_id, '_po_number', true );
if(!empty($my_var_two))
echo $my_var_two;
else
echo '<small>(<em>no value</em>)</small>';
break;
*/
}
}
// CODEMILITANT - REDIRECT WOOCOMMERCE TO THANK YOU AFTER SUCCESSFUL CHECKOUT
function cm_wc_aftersale_redirect( $order_id ){
$order = wc_get_order( $order_id );
$url = site_url() . '/thank-you/';
if ( ! $order->has_status( 'failed' ) ) {
wp_safe_redirect( $url );
exit;
}
}
add_action( 'woocommerce_thankyou', 'cm_wc_aftersale_redirect');
// CODEMILITANT TEST CODE
<?php phpinfo(); ?>
// END TEST